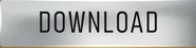
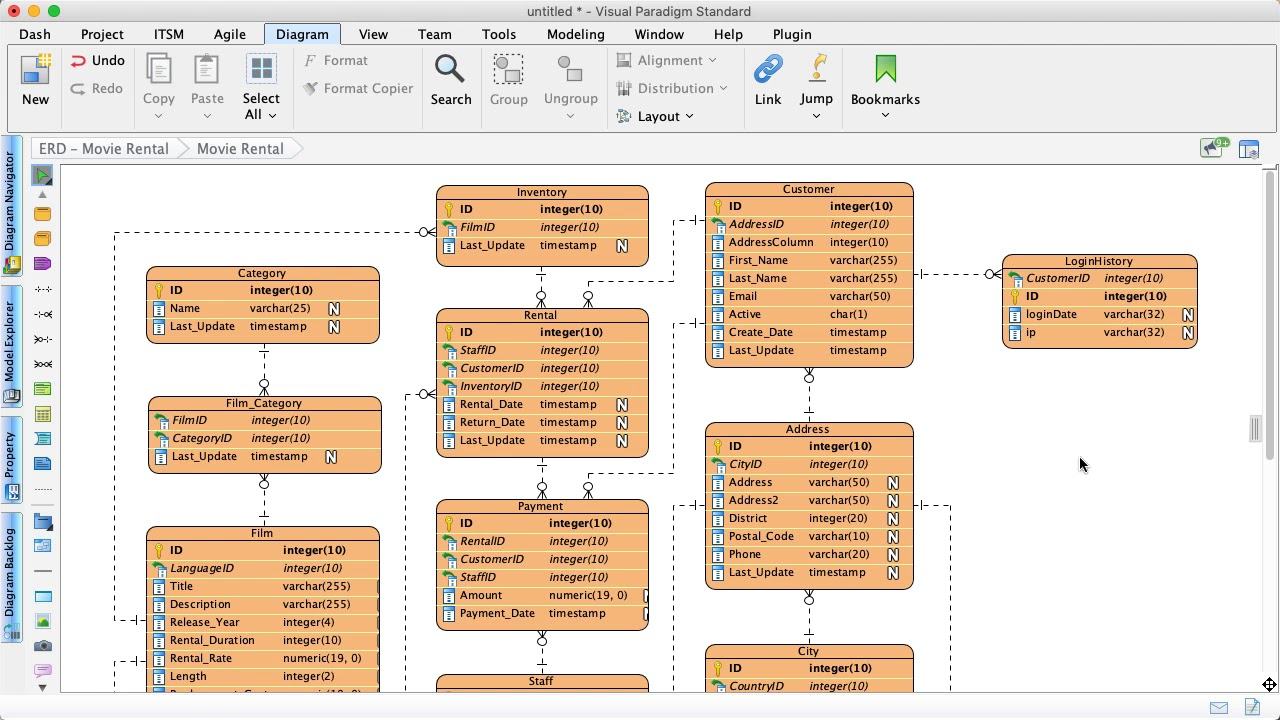
- CREATING DATABASE AND SCHEMA DB BROWSER FOR SQLITE CODE
- CREATING DATABASE AND SCHEMA DB BROWSER FOR SQLITE FREE
If the employees’ table exists, it will return its name as follows: We can also check if the table we want to access exists or not by executing the following query: cursorObj.execute('SELECT name from sqlite_master WHERE type = "table" AND name = "employees"') Similarly, to check if the table exists when deleting, we use “ if exists” with the DROP TABLE statement as follows: drop table if exists table_nameįor example, cursorObj.execute('drop table if exists projects') To check if the table doesn’t already exist, we use “ if not exists” with the CREATE TABLE statement as follows: create table if not exists table_name (column1, column2, …, columnN)ĬursorObj.execute('create table if not exists projects(id integer, name text)') Similarly, when removing/ deleting a table, the table should exist. When creating a table, we should make sure that the table is not already existed. In the above SELECT statement, instead of using the asterisk (*), we specified the id and name attributes. Now, to fetch id and names of those who have a salary greater than 800: import sqlite3ĬursorObj.execute('SELECT id, name FROM employees WHERE salary > 800.0') You can use the insert statement to populate the data, or you can enter them manually in the DB browser program.
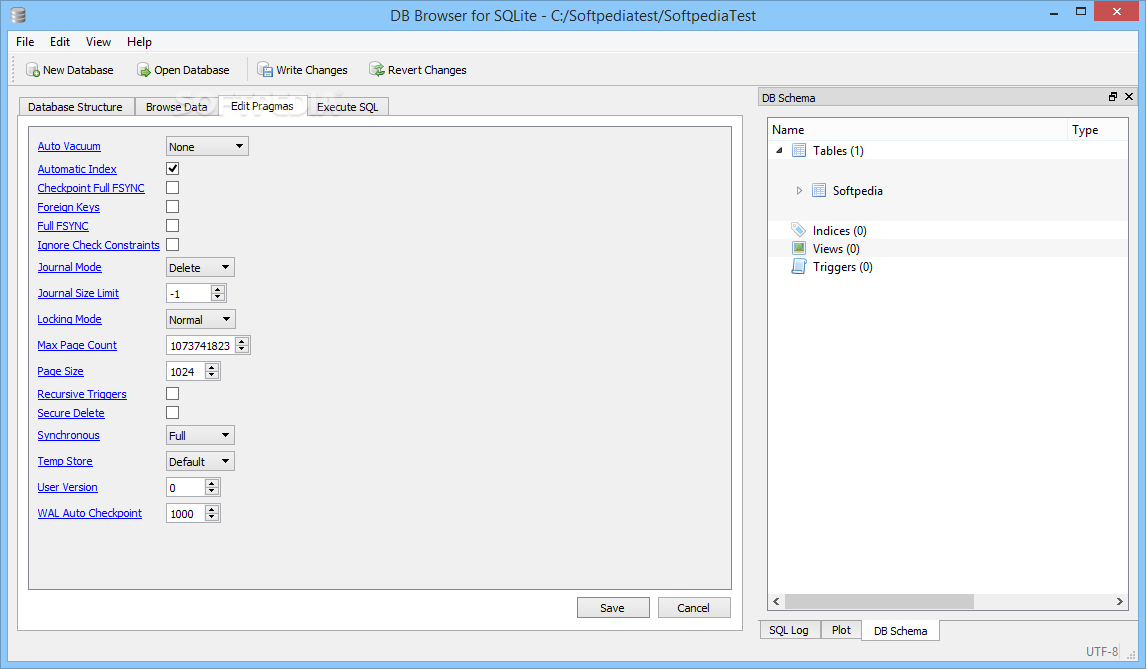
For this, let’s populate our table with more rows, then execute our query. For example, we want to fetch the ids and names of those employees whose salary is greater than 800. If you want to fetch specific data from the database, you can use the WHERE clause. You can also use the fetchall() in one line as follows:
CREATING DATABASE AND SCHEMA DB BROWSER FOR SQLITE CODE
The above code will print out the records in our database as follows: In the above code, we have defined two methods, the first one establishes a connection and the second method creates a cursor object to execute the create table statement.ĬursorObj.execute('SELECT * FROM employees') The code will be like this: import sqlite3ĬursorObj.execute("CREATE TABLE employees(id integer PRIMARY KEY, name text, salary real, department text, position text, hireDate text)") Let’s create employees with the following attributes: employees (id, name, salary, department, position, hireDate) Using the cursor object, call the execute method with create table query as the parameter.From the connection object, create a cursor object.To create a table in SQLite3, you can use the Create Table query in the execute() method.
CREATING DATABASE AND SCHEMA DB BROWSER FOR SQLITE FREE
Closing a connection is optional, but it is a good programming practice, so you free the memory from any unused resources. If there are no errors, the connection will be established and will display a message as follows.Īfter that, we have closed our connection in the finally block. Then we have except block, which in case of any exceptions prints the error message. Inside this function, we have a try block where the connect() function is returning a connection object after establishing the connection. Print("Connection is established: Database is created in memory")įirst, we import the sqlite3 module, then we define a function sql_connection. This database is called in-memory database.Ĭonsider the code below in which we have created a database with a try, except and finally blocks to handle any exceptions: import sqlite3 This database file is created on disk we can also create a database in RAM by using :memory: with the connect function. When you create a connection with SQLite, that will create a database file automatically if it doesn’t already exist. Now we can use the cursor object to call the execute() method to execute any SQL queries. To execute the SQLite3 statements, you should establish a connection at first and then create an object of the cursor using the connection object as follows: con = nnect('mydatabase.db') The SQLite3 cursor is a method of the connection object. You can create it using the cursor() method. To execute SQLite statements in Python, you need a cursor object. That will create a new file with the name ‘mydatabase.db’. You can a connection object using the connect() function: import sqlite3 To use SQLite3 in Python, first of all, you will have to import the sqlite3 module and then create a connection object which will connect us to the database and will let us execute the SQL statements.
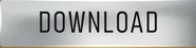